A cool way to show a items gallery is using a scrollable div as Amazon displays related books for the current selected book. This technique is known as "carousel". I made an example (Download source (85Kb)) that shows a list of motherboards using a jQuery Carousel Component populated dinamically via Ajax queries (useful when haves a large items quantity to show)
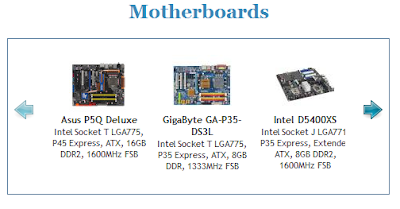
Server side pagination method
The request/response to the method that returns a "page" of the carousel are maded using JSON.
For simplicity the pagination is done against a generic collection, but easily can be a database query.
Client side pagination method
Download source: jQuery Carousel in asp.net.rar (85Kb)
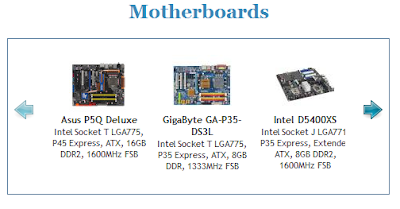
Server side pagination method
The request/response to the method that returns a "page" of the carousel are maded using JSON.
For simplicity the pagination is done against a generic collection, but easily can be a database query.
[WebMethod]
[ScriptMethod(ResponseFormat = ResponseFormat.Json)]
public static List<Motherboard> GetItems(int pageIndex, int pageSize)
{
List<Motherboard> motherboards = new List<Motherboard>
{
new Motherboard("Asus P5Q Deluxe", "Asus P5Q Deluxe Motherboard", "Intel Socket T LGA775, P45 Express, ATX, 16GB DDR2, 1600MHz FSB"),
new Motherboard("GigaByte GA-P35-DS3L", "GigaByte GA-P35-DS3L Motherboard", "Intel Socket T LGA775, P35 Express, ATX, 8GB DDR, 1333MHz FSB "),
new Motherboard("Intel D5400XS", "Intel D5400XS Motherboard", "Intel Socket J LGA771, P35 Express, Extended ATX, 8GB DDR2, 1600MHz FSB "),
new Motherboard("Asus M3N-HT Deluxe Mempipe", "Asus M3N-HT Deluxe Mempipe Motherboard", "Socket AM2+, nVIDIA nForce 780a, ATX, 8GB DDR2, 2600MHz FSB"),
new Motherboard("ABIT IP35 PRO XE", "ABIT IP35 PRO XE Motherboard", "Intel Socket T LGA775, P35 Express, ATX, 8GB DDR2, 1600MHz FSB"),
new Motherboard("Asus Striker Extreme", "Asus Striker Extreme Motherboard", "Quad-Core/Core 2 Duo Extreme/Core 2 Duo/Pentium D/Celeron D, Socket T"),
new Motherboard("XFX MB-N780-ISH9", "XFX MB-N780-ISH9 Motherboard", "Intel Socket T LGA775, nForce 780i SLI, ATX, 8GB DDR2, 1333MHz FSB "),
new Motherboard("HP Asus P5BW-LA", "HP Asus P5BW-LA Motherboard", "Intel Socket T LGA775, P965 Express, Micro ATX, 8GB DDR2, 1066MHz FSB"),
new Motherboard("Biostar P4M900-M4", "Biostar P4M900-M4 Motherboard", "Core 2 Quad/Pentium D/Pentium 4/Celeron D, Socket T SLI, ATX, 8GB DDR2, 1333MHz Bus"),
new Motherboard("MSI P6N Diamond", "MSI P6N Diamond Motherboard", "Intel Socket T LGA775, nForce 680i SLI, ATX, 8GB DDR2, 1333MHz FS"),
new Motherboard("Intel D915GAG", "Intel D915GAG Motherboard", "Pentium 4/Celeron D, Socket T, 915G, mATX, 4GB DDR, 800MHz FSB"),
new Motherboard("ABIT IP35-E", "ABIT IP35-E Motherboard", "Intel Socket T LGA775, P35 Express, ATX, 8GB DDR2, 1333MHz FSB "),
};
return motherboards.GetRange((pageIndex - 1) * pageSize, pageSize);
}
Client side pagination method
<script>
function mycarousel_makeRequest(carousel, first, last, per_page, page)
{
// Lock carousel until request has been made
carousel.lock();
// Call the server side method with pageIndex and pageSize parameters
$.ajax({
type: "POST",
url: "Default.aspx/GetItems",
data: "{'pageIndex':'" + page + "', 'pageSize':'" + per_page + "'}",
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function(data) {
mycarousel_itemAddCallback(carousel, first, last, data.d, page);
}
});
}
function mycarousel_itemAddCallback(carousel, first, last, data, page)
{
// Unlock
carousel.unlock();
// Set size
carousel.size(12);
var per_page = carousel.last - carousel.first + 1;
for (var i = first; i <= last; i++)
{
var pos = i - 1;
var idx = Math.round(((pos / per_page) - Math.floor(pos / per_page)) * per_page);
carousel.add(i, mycarousel_getItemHTML(data[idx]));
}
};
/**
* Buil item html
*/
function mycarousel_getItemHTML(mother)
{
var item = "<div>";
item += "<img src='images/" + mother.Image + ".jpg' alt='" + mother.Title + "'>";
item += "</div>";
item += "<div class='Title'>";
item += mother.Title;
item += "</div>";
item += "<div class='Description'>";
item += mother.Description;
item += "</div>";
return item;
};
</script>

1 comment:
Good job,
could you please give and example reading from the database
Post a Comment